Mastering Asynchronous Programming with JavaScript: Unleash the Power of Hapi.js in Action

In the era of high-performance web applications, managing concurrency and asynchronous tasks effectively is paramount. Node.js, a popular JavaScript runtime environment, provides a powerful platform for building such applications. Hapi.js, a robust Node.js framework, enables developers to harness the power of asynchronous programming and create scalable, responsive, and maintainable web applications.
This article delves into the intricacies of Hapi.js, exploring its features, best practices, and how it empowers developers to build robust, performant, and asynchronous web applications in JavaScript.
Asynchronous programming, a cornerstone of modern web development, involves handling multiple tasks concurrently without blocking the main event loop. This approach allows applications to remain responsive even while performing lengthy or I/O-intensive operations, such as database queries or network requests.
4.4 out of 5
Language | : | English |
File size | : | 9885 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 384 pages |
Hapi.js leverages the asynchronous nature of Node.js to provide a seamless and efficient way to manage concurrency and asynchronous tasks. It offers a comprehensive set of features and APIs designed specifically for handling asynchronous operations, enabling developers to create highly scalable and responsive applications.
Hapi.js is a powerful, open-source framework for building web applications and APIs in Node.js. Its architecture is based on the "plug-and-play" principle, allowing developers to extend its functionality by adding plugins and modules.
Hapi.js is renowned for its:
Flexibility: Allows developers to create applications tailored to their specific needs and requirements.
Extensibility: Supports a wide range of plugins and modules, providing limitless possibilities for customization.
Scalability: Designed to handle high-traffic applications with ease, ensuring optimal performance even under heavy load.
Security: Incorporates industry-standard security best practices, safeguarding applications from vulnerabilities and attacks.
To get started with Hapi.js, follow these steps:
Install Hapi.js using npm:
npm install hapi
Create a new Hapi.js server:
js const Hapi = require('hapi');
const server = Hapi.server({ host: 'localhost', port: 8000 });
- Define routes and handlers:
js server.route({ method: 'GET', path: '/', handler: (request, h) => { return 'Hello, Hapi.js!'; }});
- Start the server:
js async function start(){try { await server.start(); console.log('Server running on %s', server.info.uri); }catch (err){console.log(err); process.exit(1); }}
start();
Let's build a simple CRUD (Create, Read, Update, Delete) application using Hapi.js to illustrate its capabilities.
- Creating a Resource:
js server.route({ method: 'POST', path: '/users', handler: async (request, h) => { const newUser = request.payload; await db.createUser(newUser); return h.response().code(201); }});
- Reading Resources:
js server.route({ method: 'GET', path: '/users', handler: async (request, h) => { const users = await db.getUsers(); return users; }});
- Updating Resources:
js server.route({ method: 'PUT', path: '/users/{id}', handler: async (request, h) => { const updatedUser = request.payload; await db.updateUser(updatedUser); return h.response().code(200); }});
- Deleting Resources:
js server.route({ method: 'DELETE', path: '/users/{id}', handler: async (request, h) => { await db.deleteUser(request.params.id); return h.response().code(204); }});
To maximize the benefits of Hapi.js, follow these best practices:
Leverage Plugins and Modules: Utilize Hapi.js's extensive plugin ecosystem to enhance functionality and cater to specific application needs.
Use Error Handling Middleware: Implement error-handling middleware to catch and handle errors gracefully, preventing application crashes and ensuring a seamless user experience.
Optimize for Performance: Employ caching, pagination, and data compression techniques to improve application responsiveness and handle high traffic efficiently.
Focus on Security: Implement robust security measures, such as input validation, rate limiting, and Cross-Site Request Forgery (CSRF) protection, to safeguard applications from malicious attacks.
Hapi.js is an indispensable tool for building high-performance, scalable, and responsive web applications and APIs in JavaScript. Its asynchronous programming capabilities, extensive plugin ecosystem, and robust security features empower developers to create applications that meet the demands of modern web development.
By mastering the concepts and best practices of Hapi.js, developers can harness the power of asynchronous programming to create applications that are not only performant but also scalable, secure, and maintainable.
If you seek to delve deeper into the world of Hapi.js, I highly recommend the book "Hapi.js in Action" by Matt Harrison. This comprehensive guide provides an in-depth exploration of Hapi.js, covering everything from its core principles to advanced techniques and real-world examples. Whether you're a seasoned Node.js developer or just starting your journey, "Hapi.js in Action" is an invaluable resource that will empower you to build exceptional web applications with confidence.
4.4 out of 5
Language | : | English |
File size | : | 9885 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 384 pages |
Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Book
Novel
Page
Chapter
Text
Story
Genre
Reader
Library
Paperback
E-book
Magazine
Newspaper
Paragraph
Sentence
Bookmark
Shelf
Glossary
Bibliography
Foreword
Preface
Synopsis
Annotation
Footnote
Manuscript
Scroll
Codex
Tome
Bestseller
Classics
Library card
Narrative
Biography
Autobiography
Memoir
Reference
Encyclopedia
Michelle Corey
Matthew Reilly
Margaret Wooster
Marc Schneier
Wendy Walker
Silvia Moon
Marilyn Singer
Sherron Sparks
Mary Wood
Marie Anne Lecoeur
Marcel Dzama
Maxine Kruse
Marybeth Wuenschel
Nicola Brunswick
Summary Genie
Natalia Clarke
Richard Rushfield
Mary Kaye Asperheim
Markus Daechsel
Mark Dillon
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
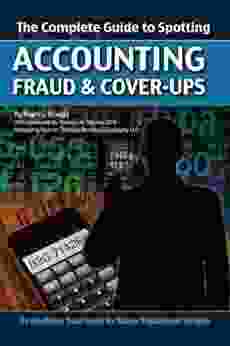

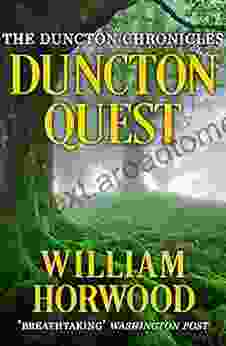

- Boris PasternakFollow ·8.2k
- Colton CarterFollow ·19.5k
- Ernesto SabatoFollow ·4.8k
- Vladimir NabokovFollow ·18.8k
- Jett PowellFollow ·5.8k
- Desmond FosterFollow ·11k
- Jack PowellFollow ·11.2k
- Robert ReedFollow ·13.6k
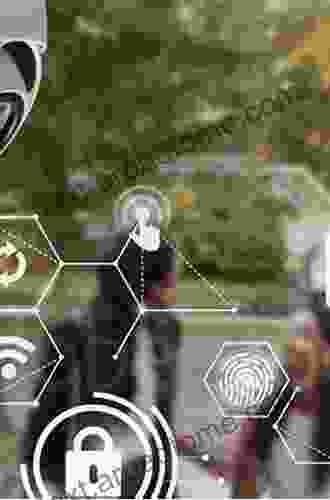

Intelligent Video Surveillance Systems: The Ultimate...
In a world...
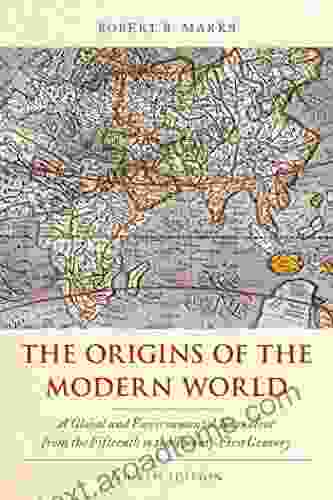

The Origins of the Modern World: A Journey to the Roots...
Embark on an Extraordinary...
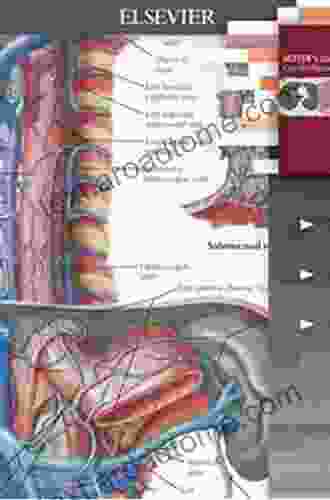

Unlock the Power of Integrated Medical Imaging with...
In the rapidly evolving...
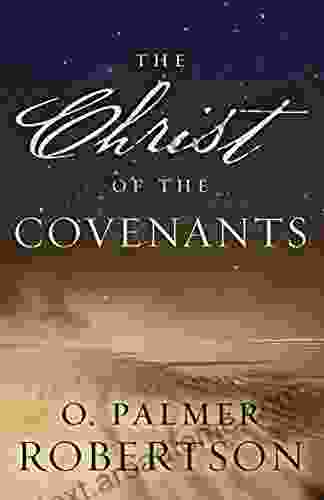

The Christ of the Covenants: Unlocking the Mystery of...
Embark on a Profound...
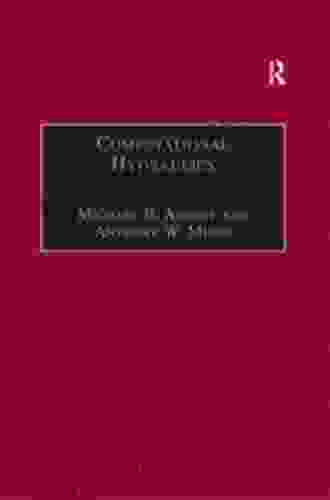

Computational Hydraulics: A Comprehensive Guide for...
In the realm of fluid dynamics,...
4.4 out of 5
Language | : | English |
File size | : | 9885 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 384 pages |